Sun Java (program ObexDemo)
Membuat Program Mengirim dan Menerima file berupa gambar
dengan Program Java
ObexDemo merupakan program untuk
mengirim dan menerima data berupa gambar menggunakan .
Alat dan Bahan :
- Sun Java (TM) Wireless Toolkit 2.5.2_01 for CLDC atau bisa gunakan yang lainnya. Untuk menginstallnya, kita harus install dulu jdk.
- Notepad++.
Langkah – Langkah :
- Kita jalankan dulu Sun Java (TM) Wireless Toolkit 2.5.2_01 for CLDC. Setelah itu, klik New Project, di ProjectName kita ketikkan nama project kita, misalnya ObexDemo, di MIDlet Class Name ketikkan tulisan yang sama, Lalu klik Create Project.
Akakan muncul kotak dialog setting,klik ok. Selanjut nya akan
ada tulisan seperti di bawah.
Creating project
“AplikasiNilai”
Ket: Disinilah tempat kita
menyimpan source atau koding program yang kita buat yaitu pada direktori SRC
Ket: Disinilah tempat kita
menyimpan file gambar atau animasi yang kita buat yaitu pada direktori RES
Ket: Disinilah tempat kita
menyimpan file fungsi yang kita buat yaitu pada direktori LIB
2.
Buka
Textpad,dan ketik source kode berikut :
A. Source GUIImageReceiver.java :
import javax.microedition.lcdui.Alert;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Form;
import javax.microedition.lcdui.Gauge;
import javax.microedition.lcdui.Image;
import javax.microedition.lcdui.ImageItem;
import javax.microedition.lcdui.Item;
import javax.microedition.lcdui.StringItem;
/**
* @version
*/
final class GUIImageReceiver implements CommandListener {
/** Shows if debug
prints should be done. */
private static
final boolean DEBUG = false;
/** DEBUG only:
keeps the class name for debug. */
private static
final String cn = "GUIImageReceiver";
/** Keeps a
reference to a parent MIDlet. */
private
ObexDemoMIDlet parent;
/** Soft button
for stopping download and returning to the mane screen. */
private Command
stopCommand = new Command("Stop", Command.BACK, 1);
/** Soft button
for returning to the main screen of demo. */
private Command
backCommand = new Command("Back", Command.STOP, 1);
/** Soft button
for confirm Image downloading. */
private Command
confirmCommand = new Command("Yes", Command.OK, 1);
/** Soft button
for reject Image downloading. */
private Command rejectCommand = new
Command("No", Command.CANCEL, 1);
/** StringItem
shows string about connection waiting state. */
private StringItem
waitingItem = new StringItem("Waiting for connection", "");
/** ImageItem
shows image on the screen */
private ImageItem
imageItem = new ImageItem("Image ...", null, Item.LAYOUT_CENTER,
null);
/** Gauge to
indicate progress of downloading process. */
private Gauge
downloadingItem = new Gauge("Downloading Image ...", false, 256, 0);
/** Form to show
current state of receiver and to show a picture. */
private Form
receiverForm = new Form("Receive Image");
/**
synchronization object to prevent downloading during permission alert.*/
private Object
alertLock = new Object();
/** Indicate that
user choose to download image or not. */
private boolean
download = false;
/** Indicate that
user has made a choose. */
private boolean
choose = false;
/** Reference to
Obex receiver part */
private
ObexImageReceiver obexReceiver = null;
/** Constructor
initialize receiverForm to show states of receiving. */
GUIImageReceiver(ObexDemoMIDlet parent) {
this.parent =
parent;
obexReceiver =
new ObexImageReceiver(this);
waitingItem.setLayout(Item.LAYOUT_CENTER);
receiverForm.append(waitingItem);
receiverForm.addCommand(backCommand);
receiverForm.setCommandListener(this);
Display.getDisplay(parent).setCurrent(receiverForm);
(new
Thread(obexReceiver)).start();
}
/**
* Responds to
commands used on this displayable.
*
* @param c
command object source of action.
* @param d screen
object containing the item the action was performed on.
*/
public void
commandAction(Command c, Displayable d) {
if (c ==
stopCommand) {
obexReceiver.stop(false);
return;
} else if (c
== backCommand) {
obexReceiver.stop(true);
parent.show();
return;
} else if (c
== confirmCommand) {
synchronized (alertLock) {
download = true;
choose
= true;
alertLock.notify();
}
} else if (c
== rejectCommand) {
synchronized (alertLock) {
download = false;
choose
= true;
alertLock.notify();
}
}
}
/** Ascs user to
download image. */
boolean
askPermission(String imageName, int imageLength) {
boolean
download = false;
// ask
permission to download image
Alert alert =
new
Alert("Connected!",
"Incoming image:\nName =
" + imageName + "\nLength = " + imageLength +
"\nWould you like to receive it ?", null, null);
alert.setTimeout(Alert.FOREVER);
alert.addCommand(confirmCommand);
alert.addCommand(rejectCommand);
alert.setCommandListener(this);
Display.getDisplay(parent).setCurrent(alert);
synchronized
(alertLock) {
if (choose
== false) {
try {
alertLock.wait();
}
catch (InterruptedException ie) {
//
don't wait then
}
}
choose =
false;
download =
this.download;
}
Display.getDisplay(parent).setCurrent(receiverForm);
return
download;
}
/**
* Shows string
with waiting for connection message.
*/
void showWaiting()
{
receiverForm.set(0, waitingItem);
receiverForm.removeCommand(stopCommand);
receiverForm.addCommand(backCommand);
downloadingItem.setValue(0);
}
/**
* Shows progress
bar of image downloading
*/
void
showProgress(int maxValue) {
downloadingItem.setValue(0);
downloadingItem.setMaxValue(maxValue);
receiverForm.set(0, downloadingItem);
receiverForm.removeCommand(backCommand);
receiverForm.addCommand(stopCommand);
}
/**
* Update progress
of image downloading
*/
void
updateProgress(int value) {
downloadingItem.setValue(value);
}
/**
* Shows
downloaded image.
*/
void
showImage(byte[] imageData) {
Image image =
Image.createImage(imageData, 0, imageData.length);
imageItem.setImage(image);
receiverForm.set(0, imageItem);
receiverForm.removeCommand(stopCommand);
receiverForm.addCommand(backCommand);
}
/**
* Shows alert
with "not ready" message.
*/
void
canNotConnectMessage() {
Alert alert =
new Alert("Warning", "Can not connect to any sender", null,
null);
alert.setTimeout(5000);
Display.getDisplay(parent).setCurrent(alert, receiverForm);
}
/**
* Shows alert
with "stop" message.
*/
void stopMessage()
{
Alert alert =
new Alert("Warning", "Sender stopped image uploading",
null, null);
alert.setTimeout(5000);
Display.getDisplay(parent).setCurrent(alert, receiverForm);
}
/**
* Stops receiving
process in OBEX part.
*/
void stop() {
obexReceiver.stop(true);
}
}
Simpan program tersebut pada direktori src seperti di atas
dengan nama GUIImageReceiver.java (nama file harus sama dengan nama kelas pada
saat kita membuat new project).
Buka new document pada TextPad (Ctrl+N)
B. Source GUIImageSender.java :
mport java.util.Vector;
import javax.microedition.lcdui.Alert;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Form;
import javax.microedition.lcdui.Gauge;
import javax.microedition.lcdui.List;
final class GUIImageSender implements CommandListener {
private static
final int TIMEOUT = 5000;
private static
final int IMAGE_COUNT = 100;
/** Keeps a
reference to a parent MIDlet. */
private
ObexDemoMIDlet parent;
/** Soft button
for stopping download and returning to the mane screen. */
private Command
stopCommand = new Command("Stop", Command.BACK, 1);
/** Soft button
for returning to the mane screen of demo. */
private Command
backCommand = new Command("Back", Command.STOP, 1);
/** Soft button
for launching an image sending. */
private Command
sendCommand = new Command("Send", Command.ITEM, 1);
/** Container of
images file names */
private Vector
imageNames;
/** List of images
titles to select a sending image */
private List
imageList;
/** Form to show
current state of sending a picture */
private Form
senderForm = new Form("Send Image");
/** Gauge to
indicate progress of downloading process */
private Gauge
sendingItem = new Gauge("", false, 256, 0);
/** Reference to
Obex sender part */
private
ObexImageSender obexSender;
/** Constructor
initialize image list and sender form */
GUIImageSender(ObexDemoMIDlet parent) {
this.parent =
parent;
setupImageList();
imageList.addCommand(sendCommand);
imageList.addCommand(backCommand);
imageList.setCommandListener(this);
senderForm.append(sendingItem);
senderForm.addCommand(stopCommand);
senderForm.setCommandListener(this);
showImageList();
}
/**
* Responds to
commands issued on "client or server" form.
*
* @param c
command object source of action
* @param d screen
object containing the item the action was performed on
*/
public void
commandAction(Command c, Displayable d) {
if (c ==
backCommand) {
parent.show();
} else if (c
== stopCommand) {
if
(obexSender != null) {
obexSender.stop();
}
showImageList();
} else if ((c
== sendCommand) || (c == List.SELECT_COMMAND)) {
obexSender
= new ObexImageSender(this);
sendImage();
} else if (c
== Alert.DISMISS_COMMAND) {
showImageList();
}
}
/**
* Shows progress
of image uploading
*/
void
showProgress(String label, int maxValue) {
sendingItem.setLabel(label);
sendingItem.setValue(0);
sendingItem.setMaxValue(maxValue);
Display.getDisplay(parent).setCurrent(senderForm);
}
/**
* Update progress
of image uploading
*/
void
updateProgress(int value) {
sendingItem.setValue(value);
}
/**
* Shows list with
image names to select one for sending to
receiver
*/
void
showImageList() {
Display.getDisplay(parent).setCurrent(imageList);
}
/**
* Shows alert
with error message
*/
void
errorMessage() {
Alert alert =
new Alert("Error", "Can't read the image", null, null);
alert.setTimeout(TIMEOUT);
alert.setCommandListener(this);
Display.getDisplay(parent).setCurrent(alert, imageList);
}
/**
* Shows alert
with "not ready" message
*/
void
notReadyMessage() {
Alert alert =
new
Alert("Warning", "Receiver isn't ready" + " to download
image", null, null);
alert.setTimeout(TIMEOUT);
alert.setCommandListener(this);
Display.getDisplay(parent).setCurrent(alert, imageList);
}
/**
* Shows alert
with "stop" message
*/
void stopMessage()
{
Alert alert = new
Alert("Warning", "Receiver terminated" + " image
loading", null, null);
alert.setTimeout(TIMEOUT);
alert.setCommandListener(this);
Display.getDisplay(parent).setCurrent(alert, imageList);
}
/** Stops Uploading
process */
void stop() {
if (obexSender
!= null) {
obexSender.stop();
}
}
/** Starts sending
process */
private void
sendImage() {
int imageIndex
= imageList.getSelectedIndex();
String
imageName = (String)imageNames.elementAt(imageIndex);
obexSender.setImageName(imageName);
(new
Thread(obexSender)).start();
}
/**
* Check the
attributes in the descriptor that identify
* images and
titles and initialize the lists of imageNames
* and imageList.
*
* The attributes
are named "ImageTitle-n" and "ImageImage-n".
* The value
"n" must start at "1" and increment by 1.
*/
private void
setupImageList() {
imageNames =
new Vector();
imageList =
new List("Select Image to send", List.IMPLICIT);
imageList.addCommand(backCommand);
imageList.setCommandListener(this);
for (int n =
1; n < IMAGE_COUNT; n++) {
String
nthImage = "ImageName-" + n;
String
image = parent.getAppProperty(nthImage);
if ((image
== null) || (image.length() == 0)) {
break;
}
String
nthTitle = "ImageTitle-" + n;
String
title = parent.getAppProperty(nthTitle);
if ((title == null) ||
(title.length() == 0)) {
title
= image;
}
imageNames.addElement(image);
imageList.append(title, null);
}
}
}
Simpan program tersebut pada direktori src seperti di atas
dengan nama GUIImageSender.java (nama file harus sama dengan nama kelas pada
saat kita membuat new project).
Buka new documen pada TextPad (Ctrl+N)
C.
Source
MIDlet.java:
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.List;
import javax.microedition.midlet.MIDlet;
/**
* @version
*/
public final class ObexDemoMIDlet extends MIDlet implements
CommandListener {
/** A list of menu
items */
private static
final String[] elements = { "Send Image", "Receive Image"
};
/** Soft button
for exiting the demo. */
private Command
exitCommand = new Command("Exit", Command.EXIT, 1);
/** Soft button
for launching a sender or receiver of images . */
private Command
startCommand = new Command("Start", Command.ITEM, 1);
/** A menu list
instance */
private final List
menuList = new List("OBEX Demo", List.IMPLICIT, elements, null);
/** A GUI part of
OBEX client which send image to server */
private
GUIImageSender imageSender = null;
/** A GUI part of
OBEX server which receive image from client */
private
GUIImageReceiver imageReceiver = null;
/** Shows that
demo was paused */
private boolean
isPaused;
public
ObexDemoMIDlet() {
menuList.setCommandListener(this);
menuList.addCommand(exitCommand);
menuList.addCommand(startCommand);
}
public boolean
isPaused() {
return
isPaused;
}
public void
startApp() {
isPaused =
false;
show();
}
public void
pauseApp() {
isPaused =
true;
}
public void
destroyApp(boolean unconditional) {
if
(imageReceiver != null) {
imageReceiver.stop();
}
if
(imageSender != null) {
imageSender.stop();
}
}
public void
commandAction(Command c, Displayable s) {
if (c ==
exitCommand) {
destroyApp(true);
notifyDestroyed();
} else if ((c
== startCommand) || (c == List.SELECT_COMMAND)) {
switch
(menuList.getSelectedIndex()) {
case 0:
imageSender = new GUIImageSender(this);
break;
case 1:
imageReceiver = new GUIImageReceiver(this);
break;
default:
System.err.println("Unexpected choice...");
break;
}
}
}
/**
* Shows main menu
of MIDlet on the screen.
*/
void show() {
Display.getDisplay(this).setCurrent(menuList);
}
}
Simpan program tersebut pada direktori src seperti di atas
dengan nama MIDlet.java (nama file harus sama dengan nama kelas pada saat kita
membuat new project).
Buka new documen pada TextPad (Ctrl+N)
D. Source ObexDemoReceiver.java :
import java.io.IOException;
import java.io.InputStream;
import javax.microedition.io.Connection;
import javax.microedition.io.Connector;
import javax.obex.HeaderSet;
import javax.obex.Operation;
import javax.obex.ResponseCodes;
import javax.obex.ServerRequestHandler;
import javax.obex.SessionNotifier;
/**
* @version
*/
final class ObexImageReceiver implements Runnable {
/** Shows if debug
prints should be done. */
private static
final boolean DEBUG = false;
/** DEBUG only:
keeps the class name for debug. */
private static
final String cn = "ObexImageReceiver";
/** List of
supported states. */
private static
final byte NONE = 0x00;
private static
final byte OPENED = 0x01;
private static
final byte CONNECTED = 0x02;
private static
final byte STARTED = 0x03;
private static
final byte STOPED = 0x4;
private static
final byte CLOSED = 0x5;
/** Max quantity
of processing senders. */
private static
final int MAX_PROCESSING_LENGTH = 2;
/** Current state
of receiver. */
private byte
current_state = NONE;
/** Handler to
process OBEX operation. */
private Handler
handler = new Handler();
/** Indicate the
length of connection request queue. */
private int
processingLength = 0;
/** Session to
receive an image. */
private
SessionNotifier session = null;
/** Put Operation
of image downloading process. */
private Operation
operation = null;
/** Input stream
of OBEX. */
private
InputStream inputStream = null;
/** Reference to
GUI part of sender */
private
GUIImageReceiver gui = null;
ObexImageReceiver(GUIImageReceiver gui) {
this.gui =
gui;
}
/**
* Used to accept
connection and download an image.
*/
public void run() {
try {
//
synchronized to prevent loss of opened session
synchronized (this) {
//String url = "tcpobex://:5010";
String
url = "irdaobex://localhost.0010;ias=ImageExchange";
session
= (SessionNotifier)Connector.open(url);
current_state = OPENED;
}
while
(current_state != CLOSED) {
session.acceptAndOpen(handler);
preventOverloading();
}
} catch
(IOException ioe) {
synchronized (this) {
if
(current_state != CLOSED) {
ioe.printStackTrace();
}
}
}
synchronized
(this) {
if
(current_state != CLOSED) {
current_state = CLOSED;
gui.canNotConnectMessage();
}
}
closeAll(true);
}
/**
* Prevents
connection more than MAX_PROCESSING_LENGTH senders.
*/
private void
preventOverloading() {
synchronized
(handler) {
while
(processingLength >= MAX_PROCESSING_LENGTH) {
processingLength++;
try {
handler.wait();
}
catch (InterruptedException ie) {
//
don't wait then
}
processingLength--;
if
(processingLength > 0) {
handler.notify();
}
}
}
}
/**
* Starts
synchronized process of image receiving.
*/
private void
startSynchReceiving(Operation operation)
throws
IOException {
synchronized
(handler) {
processingLength++;
if
(processingLength > 1) {
try {
handler.wait();
}
catch (InterruptedException ie) {
//
don't wait then
}
}
}
synchronized
(this) {
if
(current_state == CLOSED) {
throw
new IOException();
}
this.operation = operation;
current_state = CONNECTED;
}
}
/**
* Stops
synchronized process of image receiving.
*/
private void
stopSynchReceiving() {
synchronized
(handler) {
processingLength--;
if
(processingLength > 0) {
handler.notify();
}
}
}
/**
* Tries to
receive.
*/
private int imageReceive(Operation
operation) {
HeaderSet
headers = null;
String
imageName = null;
int
imageLength = 0;
int
responseCode;
try {
startSynchReceiving(operation);
//
download information about image
headers =
operation.getReceivedHeaders();
imageName
= (String)headers.getHeader(HeaderSet.NAME);
imageLength =
(int)((Long)headers.getHeader(HeaderSet.LENGTH)).longValue();
// ask
permission to download image
if
(gui.askPermission(imageName, imageLength)) {
//
download and show image
gui.showImage(downloadImage(imageLength));
responseCode = ResponseCodes.OBEX_HTTP_OK;
} else {
responseCode = ResponseCodes.OBEX_HTTP_FORBIDDEN;
}
} catch
(IOException ioe) {
if
(current_state == STOPED) {
gui.showWaiting();
closeAll(false);
responseCode = ResponseCodes.OBEX_HTTP_RESET;
} else if
(current_state == CONNECTED) {
gui.canNotConnectMessage();
closeAll(false);
responseCode = ResponseCodes.OBEX_HTTP_INTERNAL_ERROR;
} else if (current_state
== STARTED) {
gui.stopMessage();
gui.showWaiting();
closeAll(false);
responseCode = ResponseCodes.OBEX_HTTP_INTERNAL_ERROR;
} else {
// CLOSED, OPENED ?, NONE ???
synchronized (this) {
current_state = CLOSED;
}
closeAll(true);
responseCode = ResponseCodes.OBEX_HTTP_INTERNAL_ERROR;
}
}
stopSynchReceiving();
return responseCode;
}
/** Download
image. */
private byte[]
downloadImage(int imageLength) throws IOException {
byte[]
imageData = new byte[imageLength];
int position =
0;
int length =
0;
checkStopSignal();
gui.showProgress(imageLength);
synchronized
(this) {
current_state = STARTED;
inputStream = operation.openInputStream();
}
while
(position < imageLength) {
InputStream inputStream = this.inputStream;
if
(inputStream == null) {
throw
new IOException();
}
checkStopSignal();
if
(position < (imageLength - 256)) {
length
= inputStream.read(imageData, position, 256);
} else {
length
= inputStream.read(imageData, position, imageLength - position);
}
if (length
< 0) {
throw
new IOException();
}
position
+= length;
gui.updateProgress(position);
}
synchronized
(this) {
current_state = CLOSED;
}
closeAll(true);
return
imageData;
}
/** Throws
IOException if current_state is STOPED. */
private void
checkStopSignal() throws IOException {
synchronized
(this) {
if
((current_state == STOPED) || (current_state == CLOSED)) {
throw
new IOException();
}
}
}
/** Stops
downloading process. */
void stop(boolean
stopSession) {
synchronized
(this) {
if
(stopSession) {
current_state = CLOSED;
} else {
current_state = STOPED;
synchronized (handler) {
handler.notifyAll();
}
}
closeAll(stopSession);
}
}
/** Closes all
connections. */
private void
closeAll(boolean closeSession) {
synchronized
(this) {
if
(inputStream != null) {
try {
inputStream.close();
}
catch (IOException ioe) {
}
inputStream = null;
}
if
(operation != null) {
try {
operation.close();
}
catch (IOException ioe) {
}
operation = null;
}
if
((session != null) && (closeSession)) {
if
(DEBUG) {
System.out.println(cn
+ ".closeAll: closing session");
}
try {
session.close();
}
catch (IOException ioe) {
if
(DEBUG) {
System.out.println(cn + ".closeAll: got exc: " + ioe);
}
}
if
(DEBUG) {
System.out.println(cn + ".closeAll: session is closed");
}
session = null;
}
}
}
class Handler
extends ServerRequestHandler {
public
Handler() {
}
public int
onPut(Operation operation) {
return
imageReceive(operation);
}
}
}
Simpan program tersebut pada direktori src seperti di atas
dengan nama ObexImageReceiver.java (nama file harus sama dengan nama kelas pada
saat kita membuat new project).
Buka new documen pada TextPad (Ctrl+N)
E. Source ObexImageSender.java:
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import javax.microedition.io.Connector;
import javax.obex.ClientSession;
import javax.obex.HeaderSet;
import javax.obex.Operation;
import javax.obex.ResponseCodes;
final class ObexImageSender implements Runnable {
/** Shows if debug
prints should be done. */
private static
final boolean DEBUG = false;
/** Indicate that
uploading should be stopped */
private boolean
stop = true;
/** Stream with image data */
private
InputStream imageSource;
/** Special stream
to load image data to byte array */
private
ByteArrayOutputStream baos;
/** Session to
send an image */
private
ClientSession session;
/** Put Operation
of image uploading process */
private Operation
operation;
/** Output stream
of obex */
private
OutputStream outputStream;
/** Array with
image data */
private byte[]
imageData;
/** Contains name
of uploading image */
private String
imageName;
/** Reference to
GUI part of sender */
private
GUIImageSender gui;
ObexImageSender(GUIImageSender gui) {
this.gui =
gui;
}
void
setImageName(String imageName) {
this.imageName
= imageName;
}
/**
* Used to send an
image
*/
public void run()
{
boolean loaded
= false;
boolean
connected = false;
stop = false;
try {
loadImageData(imageName);
loaded =
true;
connect();
if (stop) {
throw
new IOException();
}
connected
= true;
uploadImage(imageName);
} catch
(IOException e) {
if (DEBUG)
{
e.printStackTrace();
}
if (!stop) {
if
(connected) {
gui.stopMessage();
} else
if (loaded) {
gui.notReadyMessage();
} else
{
gui.errorMessage();
}
closeAll();
return;
}
} catch
(Throwable e) {
e.printStackTrace();
}
closeAll();
gui.showImageList();
}
/** load image
data to array */
private void
loadImageData(String imageName) throws IOException {
imageSource =
getClass().getResourceAsStream(imageName);
// read image
data and create a byte array
byte[] buff =
new byte[1024];
baos = new
ByteArrayOutputStream(1024);
while (true) {
// check
stop signal
if (stop)
{
throw
new IOException();
}
int length = imageSource.read(buff);
if (length
== -1) {
break;
}
baos.write(buff, 0, length);
}
imageData =
baos.toByteArray();
}
/** Connects with
image receiver */
private void
connect() throws IOException {
//String url =
"tcpobex://localhost:5010";
String url =
"irdaobex://discover.0210;ias=ImageExchange";
// update the Gauge message before
connecting
gui.showProgress("Connecting ...", imageData.length);
session =
(ClientSession)Connector.open(url);
if (stop) {
throw new
IOException();
}
// update the
Gauge message after connecting
gui.showProgress("Uploading Image ...", imageData.length);
HeaderSet response =
session.connect(null);
int
responseCode = response.getResponseCode();
if (responseCode !=
ResponseCodes.OBEX_HTTP_OK) {
throw new
IOException();
}
}
/** Uploads image
to receiver */
private void
uploadImage(String imageName) throws IOException {
int position =
0;
// start put
operation
HeaderSet
headers = session.createHeaderSet();
headers.setHeader(HeaderSet.NAME, imageName);
headers.setHeader(HeaderSet.LENGTH, new Long(imageData.length));
operation =
session.put(headers);
outputStream =
operation.openOutputStream();
while
(position != imageData.length) {
OutputStream outputStream = this.outputStream;
int
sendLength =
((imageData.length - position) > 256) ? 256 : (imageData.length -
position);
if
(outputStream == null) {
throw
new IOException();
}
outputStream.write(imageData, position, sendLength);
position
+= sendLength;
gui.updateProgress(position);
}
outputStream.close();
int code =
operation.getResponseCode();
if (code !=
ResponseCodes.OBEX_HTTP_OK) {
throw new
IOException();
}
}
/** Stops
uploading process */
void stop() {
stop = true;
}
/** Closes all
connections */
private void
closeAll() {
imageData =
null;
if
(imageSource != null) {
try {
imageSource.close();
} catch
(IOException ioe) {
}
imageSource = null;
}
if (baos !=
null) {
try {
baos.close();
} catch
(IOException ioe) {
}
baos =
null;
}
if
(outputStream != null) {
try {
outputStream.close();
} catch
(IOException ioe) {
}
outputStream = null;
}
if (operation
!= null) {
try {
operation.close();
} catch
(IOException ioe) {
}
operation
= null;
}
if (session !=
null) {
try {
session.disconnect(null);
} catch
(IOException ioe) {
}
try {
session.close();
} catch
(IOException ioe) {
}
session =
null;
}
}
}
Simpan program tersebut pada direktori src seperti di atas
dengan nama ObexImageReceiver.java (nama file harus sama dengan nama kelas pada
saat kita membuat new project).
Selanjutnya Kita kembali lagi ke Sun Java (TM) Wireless Toolkit 2.5.2_01 for
CLDC.
1. Di
sini, kita klik Build untuk mengetahui apakah program yang kita buat sudah
benar atau belum. Jika masih terdapat kesalahan maka perhatikan kesalahannya
pada baris kesalahan dan lokasi kesalahan yang ditunjukan pada layar konsul.
Perbaiki kesalahan program tersebut hingga selesai simpan dan ulangi Build
kembali. Setelah proses build selesai jika tidak terdapat kesalahan, klik Run.
Kita bisa coba jalankan aplikasi buatan kita.
Gambar tampilan saat program dijalankan :
Ket : 1. Tampilan awal
2. Tampilan
Tampilan menu program
3. Tampilan
menu Send images,
4. Tampilan
menu client receive
5. Tampilan
receive images
Langkah selanjutnya adalah membuat Package. Untuk
menjalankan aplikasi ini di handphone kita harus membuat file .jad , file
inilah yang nantinya akan kita pindahkan ke handphone dan kita instal di
handphone.
Langkah-langkah pembuatan file .jad
pilih Project >> pilih
Package >> pilih Create Package
Maka file .jad akan tercipta, dan file tersebut disimpan di
dalam direk tori bin.
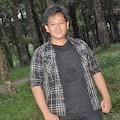
Author: Muhammad Reza Pahlevi
Related Posts:
Tugas Praktek Sistem Oprasi Tutorial
Langganan:
Posting Komentar (Atom)
0 komentar: